Blocks¶
Definition¶
A block is an entity with input and output ports, parameters and some content.
Basic blocks¶
Input block¶
Type:
basic.input
States:
FPGA pin: yellow
Virtual: green
Show clock
Wire¶
E.g.: basic FPGA input block with name Button.
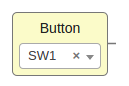
{
"data": {
"name": "Button",
"pins": [
{
"index": "0",
"name": "SW1",
"value": "10"
}
],
"virtual": false,
"clock": false
}
}
E.g.: basic Virtual input block with no name and the clock symbol.
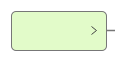
{
"data": {
"name": "",
"pins": [
{
"index": "0",
"name": "",
"value": ""
}
],
"virtual": true,
"clock": true
}
}
Bus¶
E.g.: basic FPGA input block with name in[1:0].
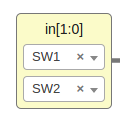
{
"data": {
"name": "in",
"range": "[1:0]",
"pins": [
{
"index": "1",
"name": "SW1",
"value": "10"
},
{
"index": "0",
"name": "SW2",
"value": "11"
}
],
"virtual": false,
"clock": false
}
}
E.g.: basic Virtual input block with name in[1:0].
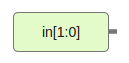
{
"data": {
"name": "in",
"range": "[1:0]",
"pins": [
{
"index": "1",
"name": "",
"value": ""
},
{
"index": "0",
"name": "",
"value": ""
}
],
"virtual": true,
"clock": false
}
}
Output block¶
Type:
basic.output
States:
FPGA pin: yellow
Virtual: green
Wire¶
E.g.: basic FPGA output block with no name.
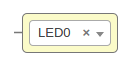
{
"data": {
"name": "",
"pins": [
{
"index": "0",
"name": "LED0",
"value": "95"
}
],
"virtual": false
}
}
E.g.: basic Virtual output block with name "out".
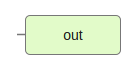
{
"data": {
"name": "out",
"pins": [
{
"index": "0",
"name": "",
"value": ""
}
],
"virtual": true
}
}
Bus¶
E.g.: basic FPGA output block with name out[1:0].
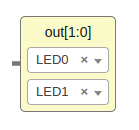
{
"data": {
"name": "out",
"range": "[1:0]",
"pins": [
{
"index": "1",
"name": "LED0",
"value": "95"
},
{
"index": "0",
"name": "LED1",
"value": "96"
}
],
"virtual": false
}
}
E.g.: basic Virtual output block with name [1:0].
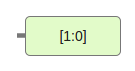
{
"data": {
"name": "",
"range": "[1:0]",
"pins": [
{
"index": "1",
"name": "",
"value": ""
},
{
"index": "0",
"name": "",
"value": ""
}
],
"virtual": true
}
}
Constant block¶
Type:
basic.constant
States:
Local parameter (lock)
E.g.: basic constant block with name value and the local flag.
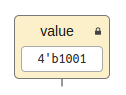
{
"data": {
"name": "value",
"value": "4'b1001",
"local": true
}
}
E.g.: basic constant block with no name and no local flag.
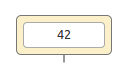
{
"data": {
"name": "",
"value": "42",
"local": false
}
}
Memory block¶
Type:
basic.memory
Address format:
Binary
Decimal
Hexadecimal
States:
Local parameter (lock)
E.g.: basic memory block with name code, binary address format and the local flag.
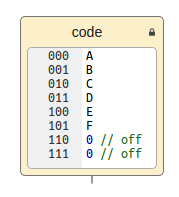
{
"data": {
"name": "code",
"list": "A \nB\nC\nD\nE\nF\n0 // off\n0 // off",
"local": true,
"format": 2
}
}
E.g.: basic memory block with no name, decimal address format and no local flag.
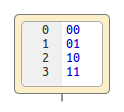
{
"data": {
"name": "",
"list": "00\n01\n10\n11",
"local": false,
"format": 10
}
}
Code block¶
Type:
basic.code
E.g.: basic code block with input port a, output port b[3:0] and parameters C and D.
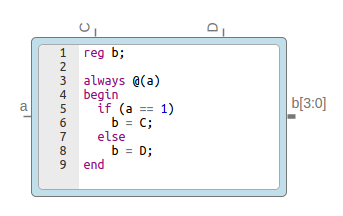
{
"data": {
"code": "reg [3:0] b_aux;\n\nalways @(a)\nbegin\n if (a == 1)\n b_aux = C;\n else\n b_aux = D;\nend\n\nassign b = b_aux;\n",
"params": [
{
"name": "C"
},
{
"name": "D"
}
],
"ports": {
"in": [
{
"name": "a"
}
],
"out": [
{
"name": "b",
"range": "[3:0]",
"size": 4
}
]
}
}
}
Information block¶
Type:
basic.info
States:
Readonly
E.g.: basic information block in editor mode.
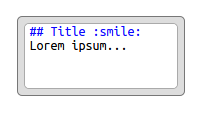
{
"data": {
"info": "## Title :smile:\nLorem ipsum...\n",
"readonly": false
}
}
E.g.: basic information block in render mode.
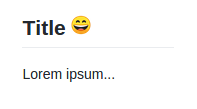
{
"data": {
"info": "## Title :smile:\nLorem ipsum...\n",
"readonly": true
}
}
Generic blocks¶
Any project can be added as a read-only generic block:
The input blocks become input ports.
The output blocks become output ports.
The constant blocks become parameters.
The block information is stored in dependencies, without the unnecessary information:
The version number is removed.
The FPGA board is removed.
The FPGA data.pins are removed.
An additional field data.size with the pins.length is created if greatter than 1.
The data.virtual flag is removed.
E.g.: this project block.ice.
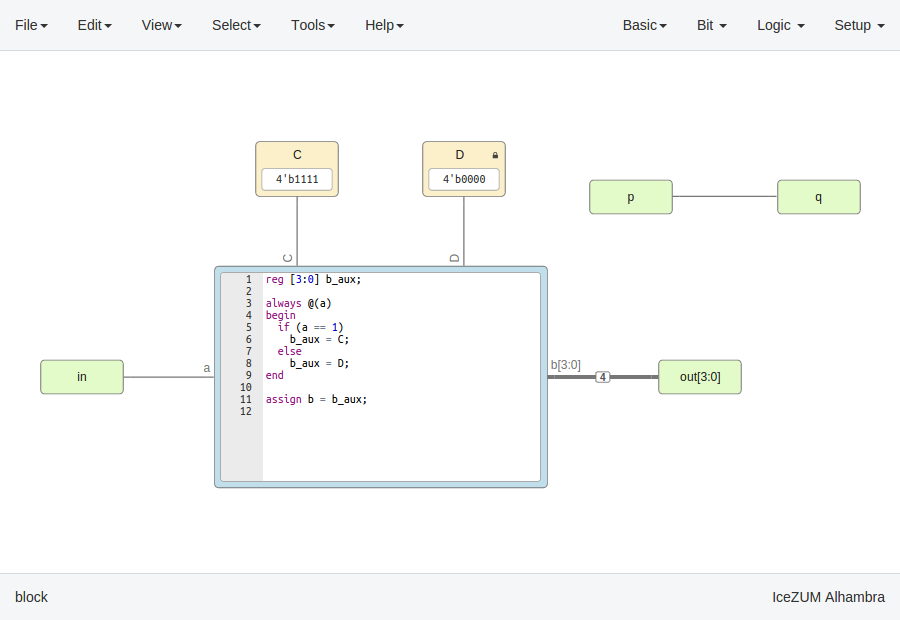
becomes this block:
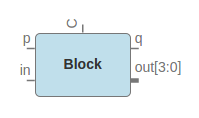
Show/Hide code
{
"version": "1.2",
"package": {
"name": "Block",
"version": "1.0",
"description": "out = in ? C : D; p = q;",
"author": "Jesús Arroyo",
"image": ""
},
"design": {
"board": "icezum",
"graph": {
"blocks": [
{
"id": "4aa2ce96-a449-42f1-b612-2c852dc50da8",
"type": "basic.input",
"data": {
"name": "p",
"pins": [
{
"index": "0",
"name": "",
"value": 0
}
],
"virtual": true
},
"position": {
"x": 680,
"y": 64
}
},
{
"id": "7f5654cf-4591-4a66-9147-d0cbdcb95f79",
"type": "basic.output",
"data": {
"name": "q",
"pins": [
{
"index": "0",
"name": "",
"value": 0
}
],
"virtual": true
},
"position": {
"x": 896,
"y": 64
}
},
{
"id": "cbd336da-6d61-4c71-90e1-e11bbe6817fc",
"type": "basic.input",
"data": {
"name": "in",
"pins": [
{
"index": "0",
"name": "",
"value": 0
}
],
"virtual": true
},
"position": {
"x": 48,
"y": 272
}
},
{
"id": "15e91005-34e6-4ce9-80b4-8c33c6c1e5a0",
"type": "basic.output",
"data": {
"name": "out",
"range": "[3:0]",
"pins": [
{
"index": "3",
"name": "",
"value": 0
},
{
"index": "2",
"name": "",
"value": 0
},
{
"index": "1",
"name": "",
"value": 0
},
{
"index": "0",
"name": "",
"value": 0
}
],
"virtual": true
},
"position": {
"x": 760,
"y": 272
}
},
{
"id": "deddfc29-7bf1-4ac4-a24e-e91b4cc14335",
"type": "basic.constant",
"data": {
"name": "C",
"value": "4'b1111",
"local": false
},
"position": {
"x": 296,
"y": 32
}
},
{
"id": "a9f85080-6523-428b-966c-359be16be956",
"type": "basic.constant",
"data": {
"name": "D",
"value": "4'b0000",
"local": true
},
"position": {
"x": 488,
"y": 32
}
},
{
"id": "fecaab8e-c7d4-4823-81fb-2b0d42f38026",
"type": "basic.code",
"data": {
"code": "reg [3:0] b_aux;\n\nalways @(a)\nbegin\n if (a == 1)\n b_aux = C;\n else\n b_aux = D;\nend\n\nassign b = b_aux;\n",
"params": [
{
"name": "C"
},
{
"name": "D"
}
],
"ports": {
"in": [
{
"name": "a"
}
],
"out": [
{
"name": "b",
"range": "[3:0]",
"size": 4
}
]
}
},
"position": {
"x": 248,
"y": 176
},
"size": {
"width": 384,
"height": 256
}
}
],
"wires": [
{
"source": {
"block": "a9f85080-6523-428b-966c-359be16be956",
"port": "constant-out"
},
"target": {
"block": "fecaab8e-c7d4-4823-81fb-2b0d42f38026",
"port": "D"
}
},
{
"source": {
"block": "deddfc29-7bf1-4ac4-a24e-e91b4cc14335",
"port": "constant-out"
},
"target": {
"block": "fecaab8e-c7d4-4823-81fb-2b0d42f38026",
"port": "C"
}
},
{
"source": {
"block": "cbd336da-6d61-4c71-90e1-e11bbe6817fc",
"port": "out"
},
"target": {
"block": "fecaab8e-c7d4-4823-81fb-2b0d42f38026",
"port": "a"
}
},
{
"source": {
"block": "fecaab8e-c7d4-4823-81fb-2b0d42f38026",
"port": "b"
},
"target": {
"block": "15e91005-34e6-4ce9-80b4-8c33c6c1e5a0",
"port": "in"
},
"size": 4
},
{
"source": {
"block": "4aa2ce96-a449-42f1-b612-2c852dc50da8",
"port": "out"
},
"target": {
"block": "7f5654cf-4591-4a66-9147-d0cbdcb95f79",
"port": "in"
}
}
]
}
},
"dependencies": {}
}